List
Description
The "List" module purpose - render list of some records from your database to work with them.
Configuration
Basic example:
To add to the page List module you need paste AlexKudrya\Adminix\Modules\List\ListModule
class instance as an argument to addModule
or addModules
method of AdminixPage
object.
ListModule
configuration
Full settings list:
Method | Description | |
---|---|---|
name | Name of module, must be unique in current page.
name('users')
| Required |
title | Title on the top of the module
title('USERS')
| Optional |
pagination | Number of items per page. To disable pagination must be equal to 0 or not exist.
pagination(20)
| Optional |
dataSource | Source of data for List, can be an
dataSource(\App\Models\User::class)
| Required |
withDeleted | Determines render or not deleted records (working with models with included By default, is not active
withDeleted()
| Optional |
editable | Determines possibility to edit records directly in the List. Editable possibility for every field determines in his personal config. By default, is not active
editable(),
| Optional |
primaryKey | Primary key in source table. Used for editable mode. Usually it is "id"
primaryKey('id')
| Required if editable mode is enabled |
criteria | Database
criteria([
['is_admin', '=', false]
])
Or you can paste parameter from route:
criteria([
['user_id', '=', 'param:1']
])
For example, for | Optional |
resource | Laravel
resource(\App\Http\Resources\UserAdminResource::class)
| Optional |
addField addFields | Methods by which you can add fields to List for displaying. Arguments can be only Detailed here #Fields
addField(
ListField::name('Name')
->field('name')
->type(ListFieldTypeEnum::STRING)
->sortable()
)
| Required |
search | Render "Search" input on the top of module. Argument -
search(
ListSearch::searchFields(['name', 'email'])
->placeholder('Search users')
)
Detailed settings described below. #Searching | Optional |
addFilter addFilters | Methods by which you can add filters for current List. Arguments can be only several classes instances:
->addFilters(
ListFilter::field('role_id')
->name('Role')
->src(
InputSelectSrc::nameField('name')
->valueField('id')
->dataSource(UserRole::class)
),
ListDateRangeFilter::field('created_at')
->name('Registration time')
->withTime()
)
Detailed settings described below. #Filters | Optional |
addAction addActions | Methods by which you can add action buttons (Links) for every item in current List module. Arguments can be only several classes instances:
addAction(
AdminixLinkModule::name('user_details')
->title('DETAILS')
->icon('bi bi-pencil')
->uri(UserPage::URI)
->params(['record:id']),
)
Detailed settings described below. #Actions | Optional |
sorting | Basic sorting for records in current List module. Argument can be only Example:
sorting(
Sorting::field('id')
->direction('asc')
)
| Optional |
newItemLink | Setting for "New Item" button rendered between Title and List, near to Search input. Format is similar to Adminix Link module.
newItemLink(
AdminixLinkModule::name('new_user_btn')
->title('NEW USER')
->icon('bi bi-person-fill-add')
->uri(NewUserPage::URI)
)
Detailed here #New item link | Optional |
Fields
Fields is an array, of fields to be rendered in the List.
To add Field to the List module you need paste AlexKudrya\Adminix\Modules\List\ListField
class instance as an argument to addField
or addFields
method of ListModule
object.
Example:
ListField
configurations
Method | Description | |||||||||
---|---|---|---|---|---|---|---|---|---|---|
name | Title of the field displayed on the top of table (inside
name('Role')
| Required | ||||||||
type | Type of rendered field, can be provided only by Available types for rendered field:
It determines data format to render, and type of input for Example:
type(ListFieldTypeEnum::SELECT)
| Required | ||||||||
field | Name of field in database or resource (if resource was provided).
field('role_id')
| Required | ||||||||
sortable | Determines the sorting possibility for current field. Can not be used to fields which provided by resource, and not exist in database table, or his name was modified. By default, is not enabled.
sortable()
| Optional | ||||||||
editable | Determines the editable possibility for current field. Can not be used to fields which provided by resource, and not exist in database table, or his name was modified. This will not be working if in To working correctly requires primary key (usually it is "id" field) in this fields list (can be By default, is not enabled.
editable()
| Optional | ||||||||
src | Required for fields with type For example, Argument can be only Example:
ListField::name('Role')
->field('role_id')
->type(ListFieldTypeEnum::SELECT)
->editable()
->src(
InputSelectSrc::nameField('name')
->valueField('id')
->dataSource(Role::class)
)
| |||||||||
addSelectRecords | Required for fields with type Arguments can be only Example:
ListField::name('Role')
->field('role_id')
->type(ListFieldTypeEnum::SELECT)
->editable()
->addSelectRecords(
SelectRecord::name('Admin')->value(1),
SelectRecord::name('Manager')->value(2),
SelectRecord::name('Seller')->value(3),
SelectRecord::name('Guest')->value(4),
)
...
|
Searching
Determines possibility to make full-text search in source table.
Example:
Method | Description | |
---|---|---|
searchFields | An array of fields which involved in the search process. The more fields, the slower but more accurate the search. Every field must be existed in main database table
searchFields(['name', 'email'])
| Required |
placeholder | A placeholder for Search
placeholder('Search users')
| Optional |
Filters
Add filters to the top of the module, near to search input. Provides the ability to filter the list by specific fields.
There are several types of filters available:
ListFilter -
AlexKudrya\Adminix\Modules\List\ListFilter
ListDateFilter -
AlexKudrya\Adminix\Modules\List\ListDateFilter
ListDateRangeFilter -
AlexKudrya\Adminix\Modules\List\ListDateRangeFilter
ListFilter
Basic filter - <select/>
input with defined options
Example:
Or
Method | Description | |||||||||
---|---|---|---|---|---|---|---|---|---|---|
name | Title of the filter
name('Role')
| Required | ||||||||
field | Name of the field used for filtering. Field must be existed in base database table
field('role_id')
| Required | ||||||||
src | Required if filter_records is empty. Has a format similar to
src(
InputSelectSrc::nameField('name')
->valueField('id')
->dataSource(\App\Models\Role::class)
),
| Required if no filterRecords() | ||||||||
filterRecords | Required if src is empty. It is an
addFilterRecords(
ListFilterRecord::name('Client')->value(1),
ListFilterRecord::name('Guest')->value(2),
ListFilterRecord::name('Manager')->value(3),
ListFilterRecord::name('Admin')->value(4),
)
| Required if no src |
ListDateFilter
Date filter - allows you to select records within a selected date.
Example:
Method | Description | |
---|---|---|
field | Name of the field used for filtering. Field must have
field('created_at')
| Required |
name | Title of the filter
name('Registration date')
| Required |
ListDateRangeFilter
Date range filter - allows you to select records within a date range.
Example:
Method | Description | |
---|---|---|
field | Name of the field used for filtering. Field must have
field('created_at')
| Required |
name | Title of the filter
name('Registration date')
| Required |
withTime | Determines whether the filter will filter by time or only by dates. By default, filter only by date, without time.
withTime()
| Optional |
filterFrom | Define "From" input. If set By default, it is enabled.
filterFrom(false)
| Optional |
filterTo | Define "To" input. If set By default, it is enabled.
filterTo(false)
| Optional |
Actions
"Actions" is an array of control buttons for every single record, to do some actions, such as "open", "edit", "delete", or wherever else you need.
Example:
To add Action buttons to List module you need paste AlexKudrya\Adminix\Modules\Link\AdminixLinkModule
or AlexKudrya\Adminix\Modules\Link\LinkModule
class instances as an argument to addAction
or addActions
method of AdminixPage
object.
Action buttons syntax is similar to Link module
Method | Description | |
---|---|---|
name | Title which will be displayed on the button
name('EDIT')
| Optional |
uri | In case
uri(UserPage::URI)
In case
uri('edit_user')
| Required |
icon | Icon class name from Bootsrap icons Example: to generate in the Link an icon
icon('bi bi-people-fill')
| Optional |
method | Type of HTTP request, can be provided only by In case In case By default, it is equal to
method(HttpMethodsEnum::POST)
| Required if not GET |
params | Technically it is optional directive, but It is list of parameters which you added to your action (usually it is "id"). When Parameters adds to your link like this: / Example:
params(['record:id'])
For record with id = 123 action link will be | Required |
criteria | Determines display or not this action button for current record. For example if you need display action button only for users without admin status, you can do it like this:
criteria([
['is_admin', '==', false]
])
or
criteria([
['deleted_at', '!=', null]
])
Attention! This directive support only | Optional |
New item link
Usually, in the admin panels, lists have the ability to add new records, and the New resource module is used for this. In order to display a link to a page with such a module in the correct place in the "list" module, there is this directive
Format is similar to Link module or Actions
Example:
Appearance examples
Here is example of List without editable mode:
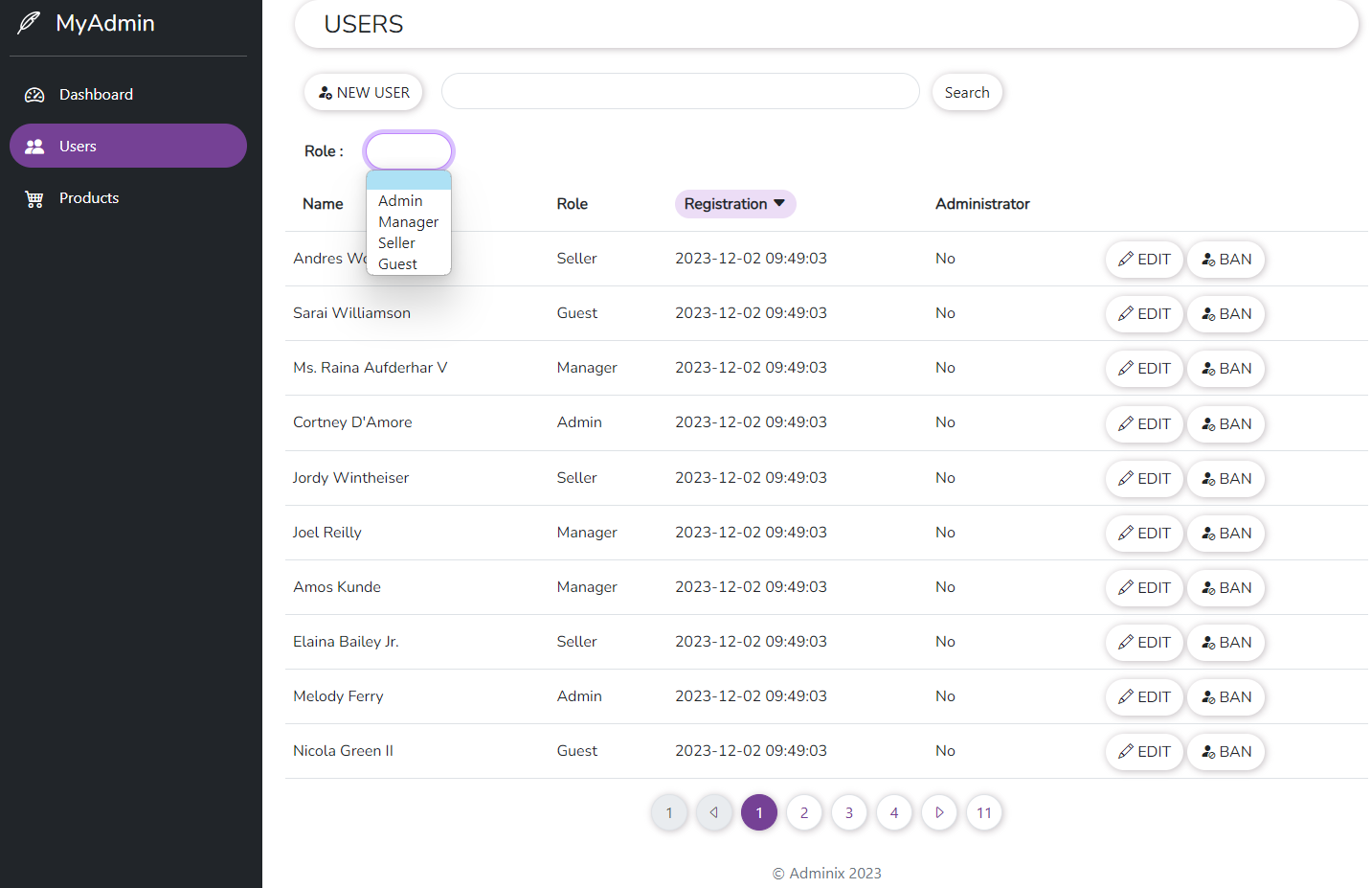
Here is example of List with editable mode:
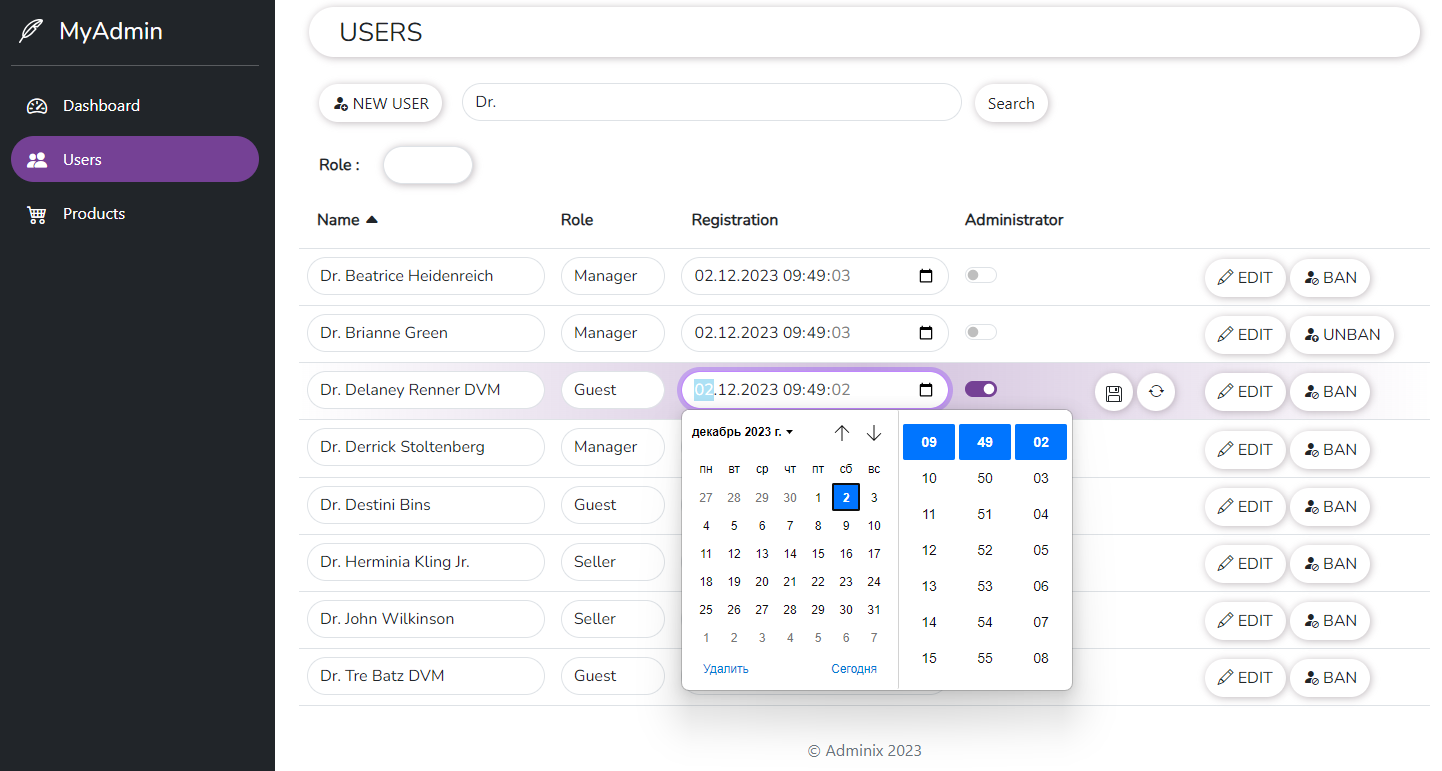